Prerequirements
- You know how to setup and configure neovim plugins.
- You use UNIX-like OS
Step-by-Step guide
- Install the next neovim plugins
-- Example with packer.nvim
use("mfussenegger/nvim-dap")
use { "rcarriga/nvim-dap-ui", requires = {"mfussenegger/nvim-dap"} }
Install vscode-lldb
- Download file specific to your system https://github.com/vadimcn/vscode-lldb/releases
- Unpack it by
unzip
utility. In my case I unpacked it to~/Sources/lldb
The result of executionunzip codelldb-x86_64-linux.vsix
in~/Sources/lldb
: - codelldb execution file is available by path:
~/Sources/lldb/extension/adapter
Configure nvim-dap in your
init.lua
local dap = require("dap")
dap.adapters.codelldb = {
type = 'server',
port = "${port}",
executable = {
-- Change this to your path!
command = '/home/kurotych/Sources/lldb/extension/adapter/codelldb',
args = {"--port", "${port}"},
}
}
dap.configurations.rust= {
{
name = "Launch file",
type = "codelldb",
request = "launch",
program = function()
return vim.fn.input('Path to executable: ', vim.fn.getcwd() .. '/', 'file')
end,
cwd = '${workspaceFolder}',
stopOnEntry = false,
},
}
require("dapui").setup({})
- Open any rust program and execute in neovim command line
:lua require("dap").toggle_breakpoint()
where you can stop your programScreenshot
- Run
:lua require("dap").continue()
in neovim command line to start debuggingScreenshot
- Run
:lua require("dapui").open()
You can navigate through debug windows by mouse.Screenshot
Execte:lua require("dapui").close()
- to close all debug windows
Keybindings
local dap = require('dap')
vim.keymap.set('n', '<F5>', function() dap.continue() end)
vim.keymap.set('n', '<F10>', function() dap.step_over() end)
vim.keymap.set('n', '<F11>', function() dap.step_into() end)
vim.keymap.set('n', '<F12>', function() dap.step_out() end)
vim.keymap.set('n', '<Leader>b', function() dap.toggle_breakpoint() end)
vim.keymap.set('n', '<Leader>dl', function() dap.run_last() end)
vim.keymap.set('n', '<Leader>df', function() require("dapui").float_element('scopes', { enter = true }) end)
Additional info
You can find at plugins repositories nvim-dap and nvim-dap-ui
dapui float_element Screenshot
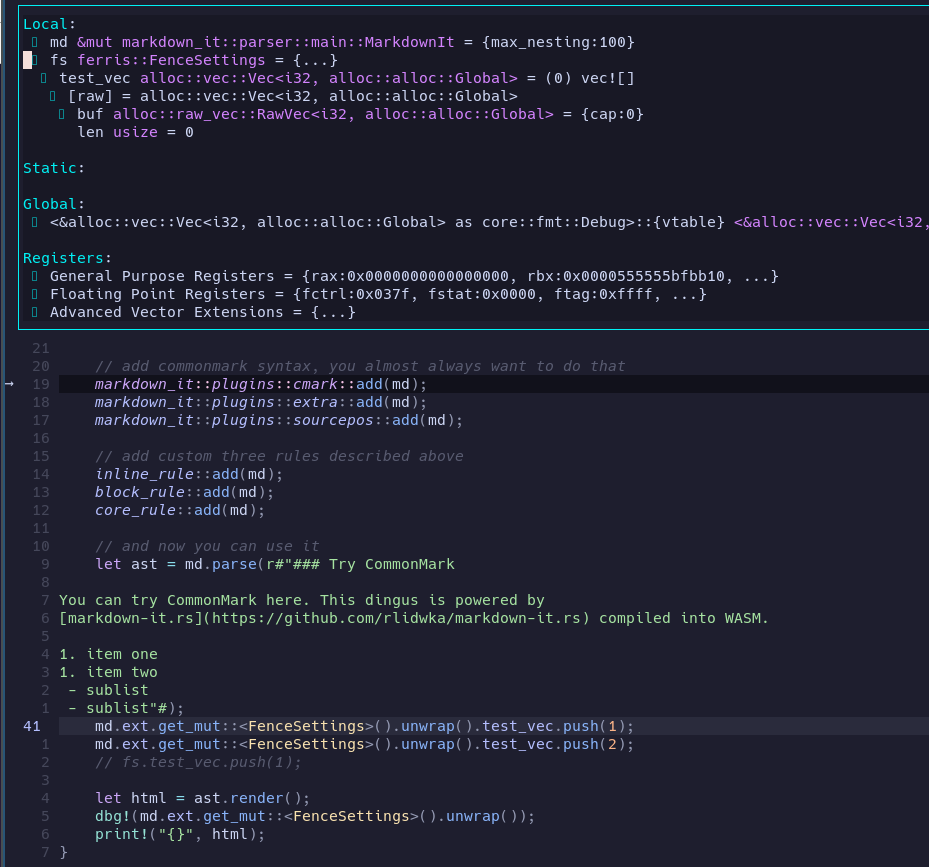